Unit testing is considered as a vital phase in software testing. Anyone who has been involved in the software development life cycle will have encountered some form of testing. Although the aim of testing is to find bugs, it cannot guarantee the absence of other faults, no matter how creative the test cases are designed. Unit testing enables a more thorough level of acceptance mechanism.
This post will give you a brief introduction on how to start with unit testing using the mock module in python.
What is Unit testing and why do we need it?
Unit testing is a type of software testing where individual units or components of a software are tested. The purpose is to validate whether each unit of the software code performs as expected. Unit tests isolate a section of code and verify its correctness.
Mock is a library for testing in Python. It helps you to replace real objects in your code with mock instances and make assertions about how they have been used.
Getting started:
Step 1. Install mock
Get started by installing the mock module. To do this simply open command prompt and type:
pip install -U mock
Step 2. Select the application you want to test.
Pick the application that you would like to test. In my case, I will be using Qxf2’s interview scheduler application. You can find it here: Interview Scheduler
Step 3. Choose a method which you want to write the unit test for:
Now, decide on the method for which you want to write the unit test. Go through the method and find out what it does.
Then choose a method call that you want to mock.
In my case, I’m choosing the is_past_date()
method from the qxf2_scheduler.py
file. It’s a simple method that tells us if the selected date is a past date or not.
def is_past_date(date): "Is this date in the past?" result_flag = True date = gcal.process_date_string(date) today = gcal.get_today() if date > = today: result_flag = False return result_flag |
Writing the Unit test
Step 1. Create a new python file to write the unit test
Ideally, you name your unit test as ‘test_‘ followed by whatever name you want. I am going to name it “test_check_past_date”
Step 2. Import the required modules
The first step in writing our unit test is to import the unit test and mock modules.
import unittest, mock
Then import the function that you would like to test. In my case, it is –
from qxf2_scheduler.qxf2_scheduler import is_past_date
I will also be importing the date parser for converting the date from string format to DateTime format before passing it to the method.
Step 3. Create a Class to hold the various unit test methods
Once you have imported the required modules, go ahead and create a class to hold the various unit test methods. Make sure to include the subclass “unittest.TestCase” while creating the test case. Your class declaration should look similar as below:
class Check_is_past_date(unittest.TestCase):
Step 4. Patch the function that you want to mock using ‘mock.patch() decorator’
The mock.patch() decorator helps us mock any function we need. In other sense, we can replicate the action performed by that function and have the function return any value of our choice. In this case, I am going to mock gcal.get_today() method and make it return a value of my choice.
I will be using the following command to mock the method:
@mock.patch('qxf2_scheduler.qxf2_scheduler.gcal.get_today',return_value=parse("2020-01-22"))
As you can see above, I have passed the path of the function to be mocked as the first argument and the return value I want as the second argument.
Step 5. Define a method for writing the test
The next step is creating a method where you’ll be writing your test conditions. The method name must start with “test_” followed by any name you want. I will name my method “test_check_if_past_date“. Pass the mock variable as an argument to the method.
Your method definition should look similar as below:
def test_check_if_past_date(self, mock_today):
Step 6. Add your required test conditions inside the method:
Add your required test scenarios inside the method. You can refer to this documentation to know more about the unit test commands.
Here, I will use the mock function to return a past date and verify if the method returns a false value.
assert is_past_date(self.date)==False
Step 7. Call the unittest in the main method
The final step is to add the main function and call the unittest from it.
if __name__=="__main__":
unittest.main()
Here is the final code:
import unittest, mock import os,sys sys.path.append(os.path.dirname(os.path.dirname(os.path.abspath(__file__)))) from qxf2_scheduler.qxf2_scheduler import is_past_date from dateutil.parser import parse class Check_is_past_date(unittest.TestCase): """ This class contains unit test for is_past_date method in qxf2_scheduler module """ #hardcoding the date date='03/04/2020' #mocking gcal.get_today() method @mock.patch('qxf2_scheduler.qxf2_scheduler.gcal.get_today',return_value=parse("2020-01-22")) def test_check_if_past_date(self, mock_today): """ This method tests whether the function returns false if the given date is not a past date """ assert is_past_date(self.date)==False if __name__=="__main__": unittest.main() |

And voila! You just ran a unit check on your application!!
I hope you found this blog helpful. Here are some other posts which I found helpful in making of this blog,
Guide to unit testing
Python unit tests using mock
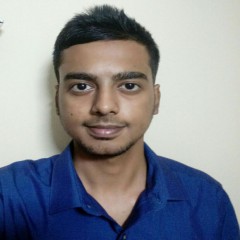
I am a QA Engineer. I completed my engineering in computer science and joined Qxf2 as an intern QA. During my internship, I got a thorough insight into software testing methodologies and practices. I later joined Qxf2 as a full-time employee after my internship period. I love automation testing and seek to learn more with new experiences and challenges. My hobbies are reading books, listening to music, playing chess and soccer.