Problem: How do I spike up my CPU load?
Recently at one of our client engagement, for one of our tests, we needed to spike up CPU usage in a controlled manner on some of our Unix servers. One way to do this was to generate a specific amount of load for a specific amount of time. This was a quick and dirty way of producing an approximate load on the CPU. This solution assumes that the CPU load from all other background processes are negligible. We came up with a simple Python script to do this and thought of sharing it with people who would be looking at something similar.
Solution
To generate a specific amount of load we will perform an arithmetic operation for that fraction of a second and then sleep for the rest. So if you want 20% utilization, the script would run the arithmetic operation for 0.2 seconds and then sleep for 0.8 seconds. Since most servers we use have multiple cores, we will automatically get the number of CPU cores and use Python’s multiprocessing module to generate load on all the cores in parallel.
""" Script to generate a specific amount of load for a specific amount of time. We will check the number of CPU cores and using multiprocessing run the generate load function on all the cores in parallel To generate a load, we will perform an arithmetic operation for a fraction of a second and then sleep for the rest So if you want 20% utilization, the script would run the arithmetic operation for 0.2 seconds and then sleep for 0.8 seconds. """ import time import math import sys import multiprocessing def generate_cpu_load(interval=int(sys.argv[1]),utilization=int(sys.argv[2])): "Generate a utilization % for a duration of interval seconds" start_time = time.time() for i in range(0,int(interval)): print("About to do some arithmetic") while time.time()-start_time < utilization/100.0: a = math.sqrt(64*64*64*64*64) print(str(i) + ". About to sleep") time.sleep(1-utilization/100.0) start_time += 1 #----START OF SCRIPT if __name__=='__main__': print("No of cpu:", multiprocessing.cpu_count()) if len(sys.argv)>2: processes = [] for _ in range (multiprocessing.cpu_count()): p = multiprocessing.Process(target =generate_cpu_load) p.start() processes.append(p) for process in processes: process.join() else: print("Usage:\n python %s interval utilization"%__file__) |
Running the Script
To run the script you need to pass the time interval you want to run the script for and the percentage of CPU utilization you want to achieve.
Eg: In case you want to increase your CPU utilization for 30 seconds by 30%, you can run the script as below
python generate_cpu_load.py 30 30 |
That was a quick way to increase your CPU usage. As a bonus, you also figured out how to use python multiprocessing. Spike up your knowledge and enjoy testing.
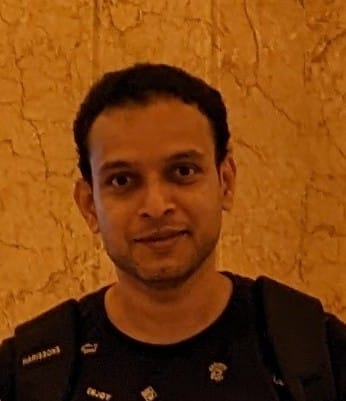
I am a dedicated quality assurance professional with a true passion for ensuring product quality and driving efficient testing processes. Throughout my career, I have gained extensive expertise in various testing domains, showcasing my versatility in testing diverse applications such as CRM, Web, Mobile, Database, and Machine Learning-based applications. What sets me apart is my ability to develop robust test scripts, ensure comprehensive test coverage, and efficiently report defects. With experience in managing teams and leading testing-related activities, I foster collaboration and drive efficiency within projects. Proficient in tools like Selenium, Appium, Mechanize, Requests, Postman, Runscope, Gatling, Locust, Jenkins, CircleCI, Docker, and Grafana, I stay up-to-date with the latest advancements in the field to deliver exceptional software products. Outside of work, I find joy and inspiration in sports, maintaining a balanced lifestyle.
Thanks Avinesh!! Good job.
Thank you Avinesh. Good script with good result