This blog is an outcome of a few adjustments that I made while moving from Java to Python when automating my tests. This will help you with basic pointers about how Python syntax is different from Java.
Why this post?
As an experienced Java automation engineer, when I started my journey into Automation using Python, I had this very basic issue in unlearning the Java syntax and adapting to new simpler syntax. I did Google and every time went to check new blog where I found part of the required syntax and by the end of my first exercise in learning Python, I had a list of syntax differences which I had to unlearn and learn. So I thought to write down those syntaxes here for someone like me.
To follow along I assume you are familiar with the Java syntax for the loops. There are quite a lot differences in the syntax so if you are used to writing braces then you will have to learn not to do that. But believe me, the syntax in Python is much easier and more understandable.
Common Syntax
1. Python is dynamically typed which means a variable that has been assigned a value of a given type may later be assigned a value of a different type. Like in the example below, variable first was int type then it is changed to string.
Example: variable_1= 2 variable_1= 'Hello' |
2. Python supports inbuilt data types like plain and long integers, Boolean, real and complex numbers.
3. It supports many types for collection of values like string, lists, and dictionaries which are very handy to use.
4. Python is line-oriented: statements end at the end of a line unless the line break is explicitly escaped with \ – I liked this a lot but believe me, I was having trouble in practicing it as it’s not how you do in Java.
5. Python comments begin with # and extend to the end of the line
6. Python strings can be enclosed in either single or double quotes (‘ or “”). Somehow I liked this one a lot. But it’s better to follow the same syntax throughout the test file.
7. There is no universally-accepted Python convention for naming classes, variables, functions, etc. But you can follow the same as which your organization follows for uniformity.
For loops and the if-else clause
1. Python supports definite looping which is nothing but the for loops, with syntax as ” for the variable in an expression”. It uses the built-in function range() with for loop as shown in the example
#(i takes on values 1, 2, 3, 4, 5, 6, 7, 8, 9) for i in range(1, 10) something to do Also, it is possible to iterate through the list # Python3 code to iterate over a list list = [1, 3, 5, 7, 9] # getting length of list length = len(list) # Iterating the index # same as 'for i in range(len(list))' for i in range(length): print(list[i]) |
2. Next is if-else condition. We write almost every function with these in it as we are trying to check some condition and do something based on the result. So say if I want to check if the URL of the page loaded by my webdriver is equal to say “https://google.com” and if it is not then I want to print something like you are not on the google page.
Java Syntax
if(driver.current_URL == "https://google.com") { print("Google page is successfully loaded. Go ahead and search"); } else { print("Sorry you are not on google page yet. Please verify your load functions"); } |
Here if you see there are curly braces within which you need to write your true and false conditions/actions and every-line is ended with a semicolon. So now below you can see same code is written in python
if(driver.current_url =="https://google.com"): print("Google page is successfully loaded. Go ahead and search") else: print("Sorry you are not on google page yet. Please verify your load functions") |
3. An interesting notation is elif condition. It is allowed as an alternative to an else. This is same as else if in java. For. example
if x > 0: something elif x < 0: something different else: yet another thing |
Hope you liked the blog. There are very simple syntactical changes but unlearning java style and adapting to this is a challenge for any experienced programmer so to start with, create your cheat sheet of the syntax which you keep messing up and I am sure in a few days of practice you will be as good as in python as you are in java.
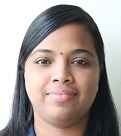
I started my career as a Dotnet Developer. I had the opportunity of writing automation scripts for unit testing in Testcomplete using C#. In a couple of years, I was given the opportunity to set up QA function for our in-house products and that’s when I became full-time Tester. I have an overall 12 years of experience. I have experience in Functional – Integration and Regression Testing, Database, Automation testing and exposure to Application Security and API testing. I have excellent experience in test processes, methodologies and delivery for products in Information security and Pharma domain. On the Personal side, I love playing with kids, watch Football, read novels by Indian Authors and cooking.