Recently, I used freezegun library to mock date for writing unit test for microservices endpoint. This is a fairly new library and I could not find many examples online. So, I wanted to blog about my experience of using this library. Freezegun library allows our Python tests to travel through time by mocking the datetime module. Once the decorator or context manager has been invoked, all calls to that particular method will return the time that has been frozen.
Use case under test:
I wanted to test a microservice endpoint ‘comments_reviewer’, this endpoint returns a message containing the names of two comment reviewers if the given date is a Thursday. On the remaining days, the endpoint will publish a message as, “No comments reviewers for today”. Based on the use case, I have written the following two test cases.
a. On a specific date, which falls on Thursday, the endpoint will display a message showing two comment reviewer’s names.
b. On other dates, a message ‘No comments reviewers’ will be shown.
I used freezegun Python library, which allowed me to freeze a particular date using freeze time decorator.
Test case:
""" Unit tests for comment reviewer's endpoint """ import main from unittest.mock import patch from messages import comments_reviewer from freezegun import freeze_time @freeze_time("2021-03-18") @patch('messages.comments_reviewer.messages',{'2021-03-18':"Today's comment reviewer's are A and B"}) def test_get_comment_reviewers(): "asserting message as per date" message = main.get_comment_reviewers() assert message['msg'] == "Today's comment reviewer's are A and B" @freeze_time("2021-03-29") @patch('messages.comments_reviewer.messages',{'2021-03-29':"No comment reviewer's for today"}) def test_no_comment_reviewers(): "asserting message as per date" message = main.get_comment_reviewers() assert message['msg']=="No comment reviewer's for today" |
After running test following output will be shown:
I hope this blog helped you to get acquainted and use this library quickly. Thanks for reading!
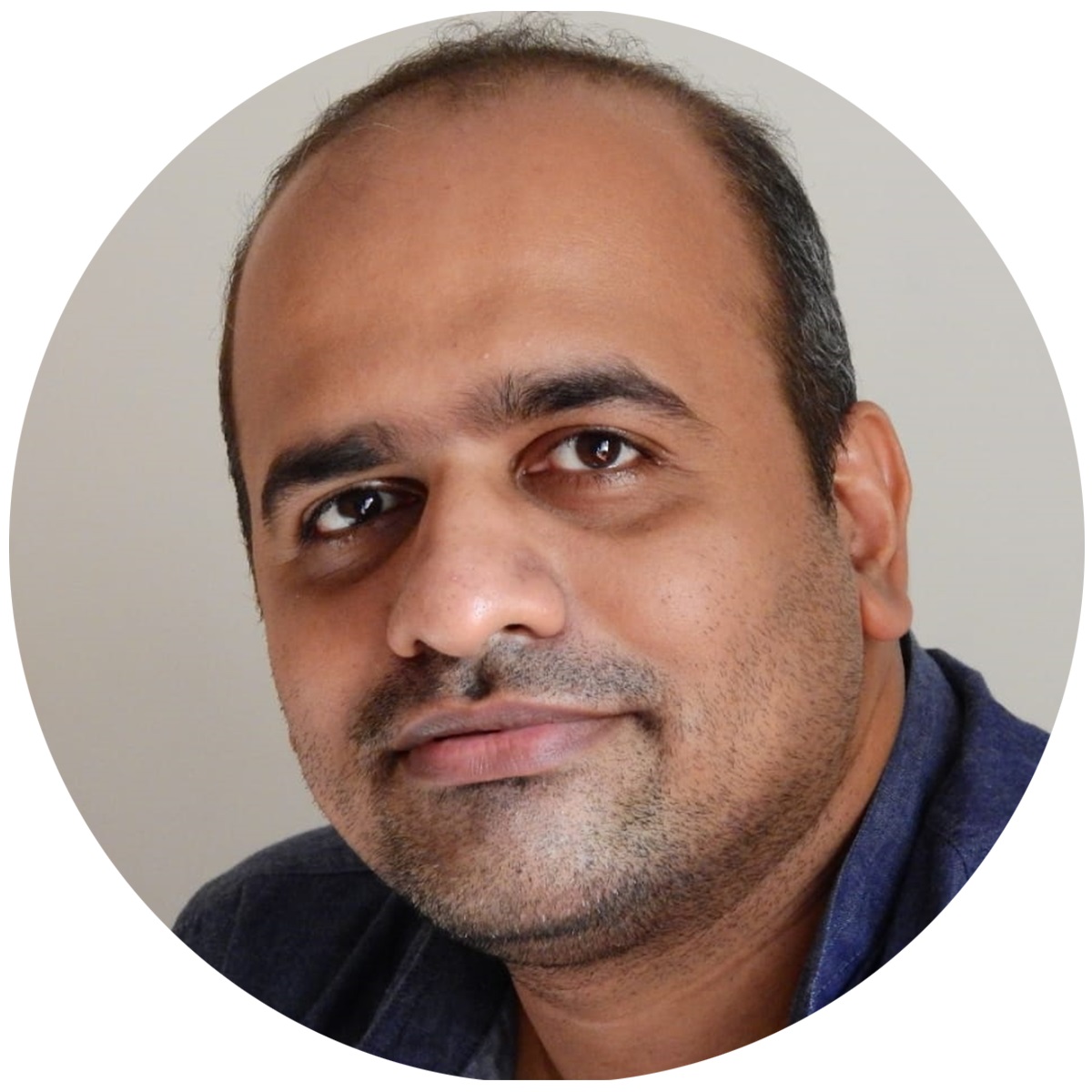
I have around 15 years of experience in Software Testing. I like tackling Software Testing problems and explore various tools and solutions that can solve those problems. On a personal front, I enjoy reading books during my leisure time.
This is a great content. This blog has in-depth information about python libraries.
Thanks for sharing