Problem: Selenium automation should run tests across different browsers and platforms on Sauce labs.
In a previous post titled Running Selenium automation using Sauce Labs: Part I, we showed you how to get started with running your Selenium tests on Sauce Labs. In this post we will show you how to parameterize the test so that you can run the test across different browsers, browser versions and operating systems. For Python newbies, we have a short section on using the Python module OptionParser.
OVERVIEW
1. Update your test class
2. Accept command line options
3. Run the test
4. Check the result on Sauce Labs
STEP 1: Update your test class
We will update the script used in Part 1 of this tutorial to include the code which accepts browser, version and platform as parameters. Update the constructor and setUp methods with the code snippet mentioned below:
class Selenium2OnSauce(unittest.TestCase): "Example class written to run Selenium tests on Sauce Labs" def __init__(self,browser,browser_version,platform): "Constructor: Accepts the browser, browser version and OS(platform) as parameters" self.browser = browser self.browser_version = browser_version self.platform = platform def setUp(self): "Setup for this test involves spinning up the right virtual machine on Sauce Labs" if self.browser.lower() == 'ff' or self.browser.lower() == 'firefox': desired_capabilities = webdriver.DesiredCapabilities.FIREFOX desired_capabilities['version'] = self.browser_version desired_capabilities['platform'] = self.platform elif self.browser.lower() == 'ie': desired_capabilities = webdriver.DesiredCapabilities.INTERNETEXPLORER desired_capabilities['version'] = self.browser_version desired_capabilities['platform'] = self.platform elif self.browser.lower() == 'chrome': desired_capabilities = webdriver.DesiredCapabilities.CHROME desired_capabilities['version'] = self.browser_version desired_capabilities['platform'] = self.platform desired_capabilities['name'] = 'Testing Script in different Browser, Version and Platform' self.driver = webdriver.Remote( desired_capabilities=desired_capabilities, command_executor="http://$USERNAME:[email protected]:80/wd/hub" ) self.driver.implicitly_wait(30) |
STEP 2: Accept command line options
Python’s OptionParser module can be used for parsing command-line options. Here is a helpful code snippet:
from optparse import OptionParser #---START OF SCRIPT if __name__ == '__main__': #Lets accept some command line options from the user #We have chosen to use the Python module optparse usage = "usage: %prog -b <browser> -v <browser version number> -p <platform>\nE.g.1: %prog -b ie -v 8 -p \"Windows 7\"\nE.g.2: %prog -b ff -v 26 -p \"Windows 8\"\n---" parser = OptionParser(usage=usage) parser.add_option("-b","--browser",dest="browser",help="The name of the browser: ie, firefox, chrome",default="firefox") parser.add_option("-v","--version",dest="browser_version",help="The version of the browser: a whole number",default=None) parser.add_option("-p","--platform",dest="platform",help="The operating system: Windows 7, Linux",default="Windows 7") (options,args) = parser.parse_args() |
STEP 3: Run the test
You can run the test script the normal way you do. We run it via the command prompt. Here is a screenshot from our machine.
STEP 4: Check the result on Sauce Labs
You can see the results on your web account. Login to your Sauce Labs account and you should see a result table like the screen shot below.
Ta-daaa! In less than 50 lines of Python, you can now get cross browser coverage for all your Selenium automated checks without having to maintain a lab.
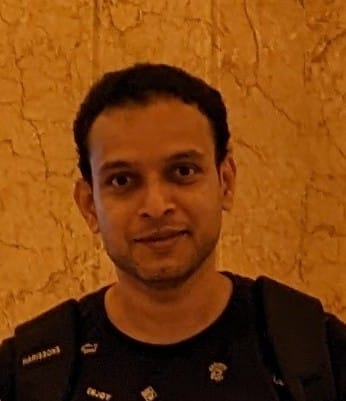
I am a dedicated quality assurance professional with a true passion for ensuring product quality and driving efficient testing processes. Throughout my career, I have gained extensive expertise in various testing domains, showcasing my versatility in testing diverse applications such as CRM, Web, Mobile, Database, and Machine Learning-based applications. What sets me apart is my ability to develop robust test scripts, ensure comprehensive test coverage, and efficiently report defects. With experience in managing teams and leading testing-related activities, I foster collaboration and drive efficiency within projects. Proficient in tools like Selenium, Appium, Mechanize, Requests, Postman, Runscope, Gatling, Locust, Jenkins, CircleCI, Docker, and Grafana, I stay up-to-date with the latest advancements in the field to deliver exceptional software products. Outside of work, I find joy and inspiration in sports, maintaining a balanced lifestyle.
One thought on “%1$s”