Problem: Many testers do not know how to identify UI elements in mobile apps.
In this post, I explain about different ways to find UI elements in Android’s calculator app. I also show you examples of using Appium’s API with the different locator strategies.
Why this post?
Identifying UI elements is a key component of writing UI automation. Most testers know how to inspect a web application and uniquely identify the UI elements that they want. However, inspecting and identifying UI elements in a mobile application is still somewhat of a dark art for most testers. Case in point – when I was learning Appium, it was hard to find a single article that showed me the various options I have to identify UI elements. This post aims to rectify this problem. In this post you will learn different ways to identify and interact with UI elements in a mobile application.
uiautomatorviewer: Mobile’s Firebug
If you automate web application testing, you are sure to have used either developer tools or Firebug. uiautomatorviewer is a GUI tool to scan and analyze the UI components of an Android app. uiautomatorviewer comes with the Android SDK. Please make sure to install Android SDK to follow along.
To launch uiautomatorviewer, pull up your command prompt, navigate to your $android-sdk/tools directory and issue the command uiautomatorviewer. Launch uiautomatorviewer and connect your Android device to your machine. Launch the calculator app. In uiautomatorviewer, click on the Device Screenshot button (top left) to take the snapshot of the calculator app. You can now inspect the layout hierarchy and view the properties of the individual UI components that are displayed on the device.
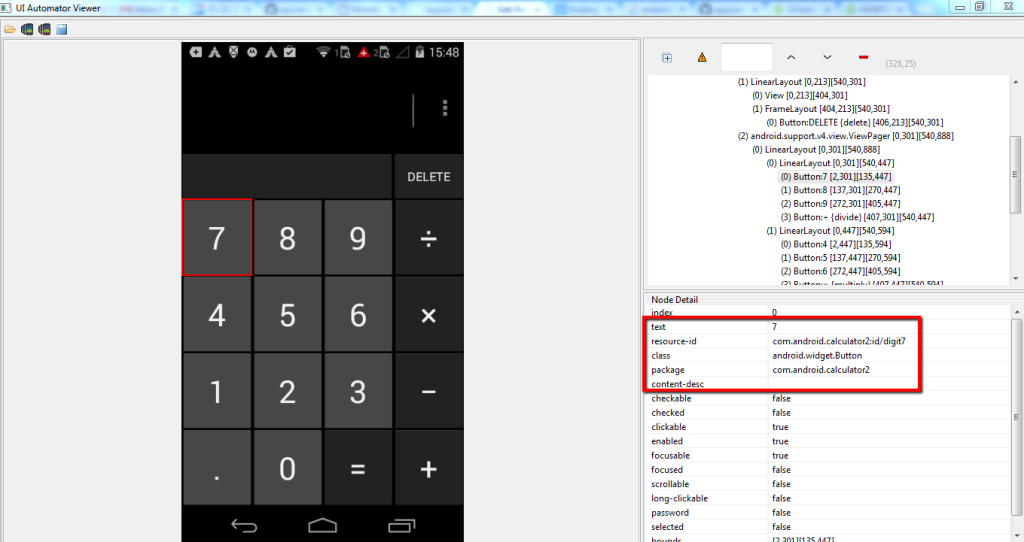
NOTE 1: For more details on connecting your Android device to your computer, please refer to the Step 2 of our previous blog.
Different ways of finding UI elements
Figure 1 is your path to happiness. You can use the properties of the elements found in the Node Detail table like text, class, resource-id, content-desc etc to find the UI components of the app. Here are a few locator strategies that map well with Appium’s API:
1. Finding element by xpath in mobile application
2. Finding mobile element by Android UIAutomator
1. Finding element by xpath in mobile applications
To write your xpath:
a. start with the class //class
b. [OPTIONAL] add attributes @attribute="blah"
E.g.: XPath to identify the number 7 in the calculator app is
//android.widget.Button[@text='7'] |
With Python and Appium, your corresponding call would be:
driver.find_element_by_xpath("//android.widget.Button[@text='7']") |
2. Finding mobile elements by Android UIAutomator
Another way to identify UI elements in a mobile application is via UiSelector. To do so,
a. start with a new UiSelector object new UiSelector()
b. dot-add any of the other properties of the element .text()
E.g.: To identify the number 7 in the calculator app:
new UiSelector().text("7") |
With Python and Appium, your corresponding call would be:
driver.find_element_by_android_uiautomator('new UiSelector().text("7")') |
NOTE: This method uses properties such as text, content-desc, resorurce id etc to find elements. Please refer to this link for more details.
Here are a few shortcuts to identifying elements that are specific to Appium:
1. Via accessibility ID: On Android, you can use the string in content-desc.
E.g.: To identify the delete button in the calculator,
driver.find_element_by_accessibility_id('delete') |
2. Finding element by Id: Corresponds to the resource-id field
E.g.: To identify the number 7 in the calculator app
driver.find_element_by_id("com.android.calculator2:id/digit7") |
3. Finding element by class name
E.g.: To find the text/results box in the calculator app
driver.find_element_by_class_name("android.widget.EditText") |
An example Appium + Python script with different locator strategies
I am providing a fully functioning Python script for you to practice your locator strategies. Challenge yourself to identify different elements in the calculator app and make corresponding changes to this script.
""" Qxf2: Example script to run one test against calculator app The test will show you how you can find UI elements by various methods like xpath, id, accessibility_id and android UIautomator on a android calculator app """ import os import unittest, time from appium import webdriver from time import sleep class Android_Calculator(unittest.TestCase): "Class to run tests for android calculator" def setUp(self): "Setup for the test" desired_caps = {} desired_caps['platformName'] = 'Android' desired_caps['platformVersion'] = '4.4' desired_caps['deviceName'] = 'Moto E' # Get the package and activity name of the calculator to launch the calculator app desired_caps['appPackage'] = 'com.android.calculator2' desired_caps['appActivity'] = 'com.android.calculator2.Calculator' self.driver = webdriver.Remote('http://localhost:4723/wd/hub', desired_caps) def tearDown(self): "Tear down the test" self.driver.quit() def test_calculator(self): "Testing android calculator" self.driver.implicitly_wait(10) # Find the UI element using xpath self.driver.find_element_by_xpath("//android.widget.Button[@text='7']").click() self.driver.find_element_by_xpath("//android.widget.Button[@resource-id='com.android.calculator2:id/mul']").click() # Find UI element using id self.driver.find_element_by_id("com.android.calculator2:id/digit7").click() # Find UI element using accessibility_id self.driver.find_element_by_accessibility_id('delete').click() # Find UI element using android UIautomator self.driver.find_element_by_android_uiautomator('new UiSelector().text("3")').click() self.driver.find_element_by_android_uiautomator('new UiSelector().resourceId("com.android.calculator2:id/equal")').click() # Find UI element using class name result=self.driver.find_element_by_class_name("android.widget.EditText") print result.get_attribute('text') #---START OF SCRIPT if __name__ == '__main__': suite = unittest.TestLoader().loadTestsFromTestCase(Android_Calculator) unittest.TextTestRunner(verbosity=2).run(suite) |
If you have read so far, you may be interested in our open-sourced Python-based mobile automation framework.Happy inspecting! Happy locating!
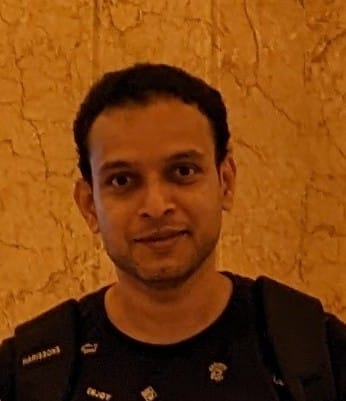
I am a dedicated quality assurance professional with a true passion for ensuring product quality and driving efficient testing processes. Throughout my career, I have gained extensive expertise in various testing domains, showcasing my versatility in testing diverse applications such as CRM, Web, Mobile, Database, and Machine Learning-based applications. What sets me apart is my ability to develop robust test scripts, ensure comprehensive test coverage, and efficiently report defects. With experience in managing teams and leading testing-related activities, I foster collaboration and drive efficiency within projects. Proficient in tools like Selenium, Appium, Mechanize, Requests, Postman, Runscope, Gatling, Locust, Jenkins, CircleCI, Docker, and Grafana, I stay up-to-date with the latest advancements in the field to deliver exceptional software products. Outside of work, I find joy and inspiration in sports, maintaining a balanced lifestyle.
Nice article.
Does Appium needs selenium for automating apps?
Can we use appium with Coded UI ?
Someone please help me on these topics.
Hi Rahul,
Appium supports a subset of the Selenium WebDriver JSON Wire Protocol, and extend it so that you can specify mobile-targeted desired capabilities to run your test through Appium.
I am not sure how to use appium on Coded UI.
Nice article
I found self.driver.find_element_by_android_uiautomator(‘new UiSelector().content-desc(“value of content description”)’).click() is not working with parameters content-desc or contentDesc ??? Can anybody tell how it works for the method find_element_by_android_uiautomator()
Sam,
Try using By.Name as mentioned in below link
http://stackoverflow.com/questions/26886800/how-to-click-on-a-button-using-content-desc
Whether the “Checkable, Checked, Clicked and enabled” node details functions can be implemented in appium mobile automation like resource-id, content – desc???? if yes please share the syntax for those functions??? Thanks in Advance
Hi,
I am not able understand what you mean by “implemented in appium”. Do you want to identify the element using “Checkable, Checked, Clicked and enabled” or get their values? Please clarify.
Hi, Can anyone please tell, for android automation testing, which tools is best to use uiautomatorviewer or appium?
NithyaDharan,
Both UIAutomator as well as Appium are good. The UIAutomatorViewer is a tool that lets you inspect elements of the app – so it complements both UIAutomator and Appium. UIAutomator is excellent for Android since it is officially supported by Android.
At Qxf2, we prefer Appium, simply because the Appium of today reminds us of the Selenium from 2006-2007. We think Appium has the potential to become the Selenium of the mobile GUI automation space. Appium is still evolving and not perfect – but like the early Selenium, it works well enough across different platforms, supports multiple languages and (while brittle) lets you write automation code that works in even the complex cases.
please help to click item which got similar name and id , by using childuiselector in python
You can use any other property which is unique to the element and find that element. Using UiSelector
a. start with a new UiSelector object new UiSelector()
b. dot-add any of the other properties of the element
E.g.: To identify the number 7 in the calculator app: new UiSelector().text(“7”)
Hi,
I opened the UIautomatorViewer by double clicking on uiautomatorviewer.bat. Then I got Ui Automator Viewer. Then I click on Device Screenshot icon. But not able to inspect individual element on the page. Only whole page identifying.
Could you please suggest how to proceed
Hi,
You should be able to inspect the individual elements on that page unless it a NAF element. Please refer https://qxf2.com/blog/naf-issue-mobile-testing/
Hi
I am also having same issue, Only whole page identifying .with one app on which i can identify all elements through and this issue only comes in appium.
So Could you please suggest whether there is some other reason fro this?
Hi Prathyusha,
I am not able to understand your issue.
My understanding is, You are not able to identify elements in Appium and you are able to identify them when you use another tool?
If Yes, what other tool are you using?
Nice article Avinash. This helped me a lot.
Using UIautomator we can find many properties of an element in mobile apps but is there any way we can find out the id of an element using any tools?
Hi Krishna,
Good to know the blog helped you.
You can also use appium inspector to find the properties of an element on the page. However for android application the appium inspector didnt work well for me. It works very well for iOS though.
If any other tool available in market same as uiautomator tool?
Hi Kamalanathan,
I am guessing that you are asking us if there is any other tool similar to uiautomatorviewer(since this post is about identifying elements) and not the uiautomator tool.
Appium inspector is another tool that can be used to identify elements in mobile apps
Is there any way to validate that I am creating a right xpath using UI Automator like we can validate the xpath in consol on web without even implementing it in our code.
Hi Gaurav,
I don’t think there is any tool that would let you do that, at the moment. We use UI Automator Viewer, Appium Inspector and XCUITest to identify UI elements for testing iOS and Android apps at Qxf2 and I am certain those three don’t let to validate your locators. Please let us know if you manage to find any tool that allows locator verification.