In a previous post, we showed you how to get started with running your Selenium automation on BrowserStack. In this post Team Qxf2 will show you how to modify your existing automation scripts to run on different platforms, browsers and devices. Since a lot of our readers seem to like mobile automation, we have chosen to run these tests on the iPhone 7 and Google Nexus 6.
Note: BrowserStack has a free trial that requires only a email to sign up. We encourage testers to take advantage of the free trial.
OVERVIEW
1. Update your test class
2. Accept command line options
3. Run the test
4. Check the result on BrowserStack
STEP 1: Update your test class
We had previously written a Selenium script to visit Chess.com, assert the title page and then click on Sign up button. If you have not seen it before, we recommend you quickly scan the script used. In this post, we will update the same script to accept platform, browser name and device as parameters. To parameterize the browser, platform and device, we will update the constructor and the setUp methods of our script with the code snippet mentioned below:
class SeleniumOnBrowserStack(unittest.TestCase): "Example class written to run Selenium tests on BrowserStack" def __init__(self, platform, realMobile, browserName, device): #Constructor: Accepts the platform, realMobile, browserName and device as parameters #realMobile if set as true will run the test in a real mobile device. self.platform = platform self.realMobile = realMobile self.browserName = browserName self.device = device def setUp(self): desired_capabilities = {'platform': self.platform, 'realMobile': 'true', 'browserName': self.browserName, 'device': self.device, 'browserstack.debug': 'true' } print 'Desired Capabilities', desired_capabilities self.driver = webdriver.Remote(command_executor='http://USERNAME:[email protected]:80/wd/hub',desired_capabilities=desired_capabilities) |
Remember to update the script with your BrowserStack USERNAME:ACCESS_KEY.
STEP 2: Accept command line options
Python’s OptionParser module can be used for parsing command-line options. Here is a helpful code snippet:
from optparse import OptionParser #---START OF SCRIPT if __name__ == '__main__': #Lets accept some command line options from the user #We have chosen to use the Python module optparse usage = "usage: %prog -p -r -b -d \nE.g.1: %prog -p iOS -r true -b safari -d 'iPhone 7'" parser = OptionParser(usage=usage) parser.add_option("-p","--platform",dest="platform",help="The name of the platform: ie, MAC, ANDROID",default="MAC") parser.add_option("-r", "--real_Mobile", dest="realMobile", help="Want to test on real Mobile: ie, true, false", default="true") parser.add_option("-b","--browserName",dest="browserName",help="The browser: ie, iPhone, android ",default="iPhone") parser.add_option("-d","--device",dest="device",help="The device name: iPhone 7, Google Nexus 6",default="iPhone 7") (options,args) = parser.parse_args() |
STEP 3: Run the test
Bringing everything together, your script should look something like the code below
import unittest, time, sys, optparse from optparse import OptionParser from selenium import webdriver from selenium.webdriver.common.desired_capabilities import DesiredCapabilities class SeleniumOnBrowserStack(unittest.TestCase): "Example class written to run Selenium tests on BrowserStack" def __init__(self,platform, browserName,device): "Constructor: Accepts the platform, browserName and device as parameters" self.platform = platform self.browserName = browserName self.device = device def setUp(self): desired_capabilities = {'platform': self.platform, 'realMobile': 'true', 'browserName': self.browserName, 'device': self.device, 'browserstack.debug': 'true' } print 'Desired Capabilities', desired_capabilities self.driver = webdriver.Remote(command_executor='http://USERNAME:[email protected]:80/wd/hub',desired_capabilities=desired_capabilities) def test_chess(self): #"An example test: Visit chess.com and click on sign up link" # Go to the URL self.driver.get("http://www.chess.com") # Assert that the Home Page has title "Chess.com - Play Chess Online - Free Games" self.assertIn("Chess.com - Play Chess Online - Free Games", self.driver.title) # Identify the xpath for Play Now button which will take you to the sign up page elem = self.driver.find_element_by_xpath("//a[@title='Play Now']") elem.click() time.sleep(5) # Print the title of sign up page print self.driver.title def tearDown(self): self.driver.quit() if __name__ == '__main__': #Lets accept some command line options from the user #We have chosen to use the Python module optparse usage = "usage: %prog -p -r -b -d \nE.g.1: %prog -p MAC -r true -b safari -d 'iPhone 7'\nE.g.2: %prog -p ANDROID -r true -b android -d 'Google Nexus 6'\n---" parser = OptionParser(usage=usage) parser.add_option("-p","--platform",dest="platform",help="The name of the platform: ie, MAC, ANDROID",default="MAC") parser.add_option("-r", "--real_Mobile", dest="realMobile", help="Want to test on real Mobile: ie, true, false", default="true") parser.add_option("-b","--browserName",dest="browserName",help="The browser: ie, iPhone, android ",default="iPhone") parser.add_option("-d","--device",dest="device",help="The device name: iPhone 7, Google Nexus 6",default="iPhone 7") (options,args) = parser.parse_args() #Create a test obj with our parameters test_obj = SeleniumOnBrowserStack(platform=options.platform,browserName=options.browserName,device=options.device) #We are explicitly calling setup and tearDown because what we are showing is technically not a unit test test_obj.setUp() #Run the test itself. NOTE: This is NOT a unit test test_obj.test_chess() #We are explicitly calling setup and tearDown because what we are showing is technically not a unit test test_obj.tearDown() |
You can run the test script the normal way you do. We like executing the script via the command prompt. Here is a screenshot from our machine.
STEP 4: Check the result on BrowserStack
You can see the results on your web account. Login to your BrowserStack account and you should see a result as shown in the screen shot below.
There you go! In less than 50 lines of Python, you can now get cross browser coverage for all your Selenium automated checks without having to maintain a lab.
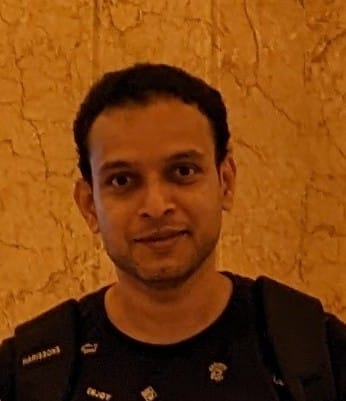
I am a dedicated quality assurance professional with a true passion for ensuring product quality and driving efficient testing processes. Throughout my career, I have gained extensive expertise in various testing domains, showcasing my versatility in testing diverse applications such as CRM, Web, Mobile, Database, and Machine Learning-based applications. What sets me apart is my ability to develop robust test scripts, ensure comprehensive test coverage, and efficiently report defects. With experience in managing teams and leading testing-related activities, I foster collaboration and drive efficiency within projects. Proficient in tools like Selenium, Appium, Mechanize, Requests, Postman, Runscope, Gatling, Locust, Jenkins, CircleCI, Docker, and Grafana, I stay up-to-date with the latest advancements in the field to deliver exceptional software products. Outside of work, I find joy and inspiration in sports, maintaining a balanced lifestyle.
HI can you guide me or provide me link for Selenium Java code
Hi vikas,
You can refer this link for the Selenium Java code: https://twitter.com/Qxf21/status/658301440906035201.
Thank you.