Suppose you are running a long Selenium test. The test runs fine but fails towards the end as some element was not found or was not clickable. Ideally, you would like to fix the locator and check it immediately. Now you wonder if you can reuse your browser session from the same point where the failure occurred. This post shows you how to do just that! This would help you to debug the locators used quickly, instead of running your whole test again.
Extract session_id and _url from driver object
We are taking advantage of the fact that Selenium lets you attach a driver to a session id and an URL. You can extract your session id and _url from the driver object as mentioned below
session_url = driver.command_executor._url session_id = driver.session_id |
Tie it up with your framework
In case you are using a framework, you can print out the session id and url in the method where you try to get the element. In an example shown below, I am using a get_element method which would return the DOM element. I am printing the session id and url whenever the find_element method fails
def get_element(self,xpath): "Return the DOM element of the xpath or 'None' if the element is not found " dom_element = None try: dom_element = self.driver.find_element(xpath) except Exception,e: self.write(str(e),'debug') self.write("Check your locator-'%s"%xpath) #Print the session_id and _url in case the element is not found self.write("In case you want to reuse session, the session_id and _url for current browser session are: %s,%s"%(driver.session_id ,driver.command_executor._url)) return dom_element |
Use the session id and url
Now you can use the session id and url, in order to debug the locators. Open python command line tool and use the below code
from selenium import webdriver #Pass the session_url you extracted driver = webdriver.Remote(command_executor=session_url,desired_capabilities={}) #Attach to the session id you extracted driver.session_id = session_id #Resume from that browser state elm = driver.find_element_by_xpath("xpath") elm.click() #Perform required action |
Note1: Currently I am able to reuse the session extracted only from Chrome browser. For Firefox there are some Issue. Link 1 and Link 2
Note2: This code is present in our open-sourced Python-Selenium test automation framework based on the page object pattern. We strongly recommend you check it out!
If you are a startup finding it hard to hire technical QA engineers, learn more about Qxf2 Services.
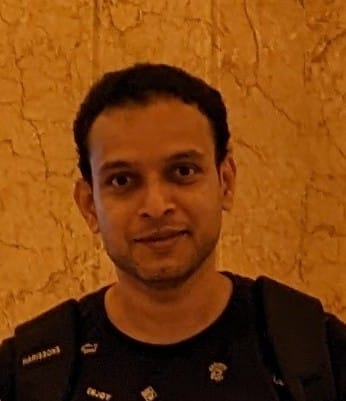
I am a dedicated quality assurance professional with a true passion for ensuring product quality and driving efficient testing processes. Throughout my career, I have gained extensive expertise in various testing domains, showcasing my versatility in testing diverse applications such as CRM, Web, Mobile, Database, and Machine Learning-based applications. What sets me apart is my ability to develop robust test scripts, ensure comprehensive test coverage, and efficiently report defects. With experience in managing teams and leading testing-related activities, I foster collaboration and drive efficiency within projects. Proficient in tools like Selenium, Appium, Mechanize, Requests, Postman, Runscope, Gatling, Locust, Jenkins, CircleCI, Docker, and Grafana, I stay up-to-date with the latest advancements in the field to deliver exceptional software products. Outside of work, I find joy and inspiration in sports, maintaining a balanced lifestyle.
I am trying to implement this however i am not able to find the solution can anyone help me with this
Can you please let us know what exact error you have hit?
Hello, thank you very much for the post, I have followed the steps to be able to reuse the browser, I am using selenium 4.19.0
options = Options()
options.add_argument(“–disable-notifications”)
options.add_argument(‘–no-sandbox’)
options.add_argument(‘–disable-dev-shm-usage’)
options.add_argument(‘–enable-logging’)
options.add_argument(‘–disable-blink-features=AutomationControlled’)
options.add_experimental_option(“detach”, True)
driver = webdriver.Chrome(options=options)
print(“ID: “, driver.session_id )
print(“URL:”, driver.command_executor._url)
When I get the id and the url I use it to reuse the browser with the following, (Example http://localhost:58822 is what driver.command_executor._url gives)
driver = webdriver.Remote(command_executor=’http://localhost:58822′, options=options)
driver.close()
driver.quit()
driver.session_id = session_id
But it is giving me the following error (I have tried with localhost, 127.0.0.1, local ip)
Ocurrió un error: HTTPConnectionPool(host=’127.0.0.1′, port=58822): Max retries exceeded with url: /session (Caused by NewConnectionError(‘: Failed to establish a new connection: [Errno 61] Connection refused’))
Has it ever happened to you? I take back my thanks
Hi,
driver.close()
&driver.quit()
statements before assigning the old session id to the new driver could be causing the exception. Can you remove those statements and try?Thanks for your response, I tried to delete it
driver.close()
driver.quit()
and I still get the same error 🙁
Can you please share the full error and the steps you are using?
Of course the complete error is
HTTPConnectionPool(host=’localhost’, port=50974): Maximum retries exceeded with URL: /session (caused by NewConnectionError(‘: Could not establish a new connection: [ Errno 61] Connection refused’))
the steps as explained above
first I generate the browser like this
options = Options()
options.add_argument(“–disable-notifications”)
options.add_argument(‘–no-sandbox’)
options.add_argument(‘–disable-dev-shm-usage’)
options.add_argument(‘–enable-logging’)
options.add_argument(‘–disable-blink-features=AutomationControlled’)
options.add_experimental_option(“detach”, True)
driver = webdriver.Chrome(options=options)
print(“ID: “, driver.session_id )
print(“URL:”, driver.command_executor._url)
then I get that session id and url to connect to the existing browser with another function
driver = webdriver.Remote(command_executor=session_url, options=options)
driver.close()
driver.quit()
driver.session_id = session_id
Hi Roberth,
Can you please make sure your script has similar code to save a session details to use it further to reconnect to the existing browser:
session_url = driver.command_executor._url
session_id = driver.session_id
And Could you give it a try with replacing
webdriver.Remote
toRemoteWebDriver
.Also please reorder
driver.session_id = session_id
line abovedriver.close()
line.Thank you for your response, yes I have reviewed it and only in the provided code I made the assignments and also adjusted the following code
driver.session_id = session_id
driver.close()
Could it be because of the version of selenium that I am using?
the version is
selenium==4.19.0
I was able to reuse the
Selenium
session by removingdriver.close()
&driver.quit()
from your snippet. I used Selenium==4.19.0.Here is my script https://gist.github.com/shivahari/f379fbe48681d78a65e3197ffdbf97e2
Hello, sorry for the recent response, I have tried the code that you have provided me and it is not the same, I still get the same error.
urllib3.exceptions.MaxRetryError: HTTPConnectionPool(host=’localhost’, port=59311): Max retries exceeded with url: /session/892e8bacd4d8674ee98a72981e39987d/screenshot (Caused by NewConnectionError(‘: Failed to establish a new connection: [Errno 61] Connection refused’))
It will be the version of python, I use Python 3.9.6.
and again I reiterate my thanks for trying to find the error
Hi,
I notice a disparity in the
URI endpoint
against which you have hitHTTPConnectionPool
exception.In the first snippet the error is seen against
/session
endpoint and in the second snippet it is against/session/$sessionId/screenshot
endpoint.These endpoints are hit at two different method calls. Calling
driver.start_session
method makes aPOST
request against/session
endpoint and callingdriver.get_screenshot_as_png
makes aGET
request against/session/$sessionId/screenshot
.The error in the second snippet you have attached
urllib3.exceptions.MaxRetryError: HTTPConnectionPool(host=’localhost’, port=59311): Max retries exceeded with url: /session/892e8bacd4d8674ee98a72981e39987d/screenshot (Caused by NewConnectionError(‘: Failed to establish a new connection: [Errno 61] Connection refused’))
proves that the session is being reused?