At Qxf2, we recently explored a tool called Replay, and got a chance to play with some of it’s features and get a feel of the tool as a whole. For starters Replay.io is a tool designed to help developers or testers record, replay, and debug browser sessions by capturing every interaction and event that occurred in the session. For QA teams, it’s a helpful tool when developers struggle to debug an issue. You can use Replay to record and share the sessions, making it easier for them to troubleshoot.
While Replay provides native support for JavaScript based frameworks like Cypress, there is very little to no information about how we could use this tool with other programming languages. So, in this blog, we will take you through setting up Replay to run with your Python Selenium tests.
1. Setting Up Replay.io
a. Generating the API key
Before you can start using Replay, you’ll need to sign up for an account on their website. Once you’ve completed the registration process, navigate to your account settings to generate an API key.
This key will be used to authenticate your requests and upload your replays to the Replay.io server.
b. Installing Replay on Ubuntu (WSL)
We first tried running Replay on Windows. However, we were hit with the realization that replay does not support Windows machines, so we had to set it up on WSL. Installing Replay is straightforward process. You can follow these steps:
Update your package lists:
sudo apt-get update |
Install Replay.io using npm:
npm i -g replayio |
Verify the installation:
replayio info |
It should show you the version of Replay that you are using.
c. Setting the API key.
We can now set the API key that we generated earlier. To do this, create an environment variable REPLAY_APP_API_KEY
and set the API key as it’s value
export REPLAY_APP_API_KEY=<Your-API-Key> |
2. Integrating Replay with Selenium tests in Python
Now, let’s take a look at how we can integrate Replay with your Selenium tests.
a. For this, we will first write a simple test that navigates to Qxf2’s selenium tutorial page and verifies the title of the page. (Note: This code is written solely for demonstration purposes for this blog post. Qxf2 does not use this approach in client projects).
"""' This is a simple test to demonstrate integration of Replay with Selenium """ from selenium import webdriver driver = webdriver.Chrome() def test_title_selenium_tutorial(): """ This is an sample automated test to check if the title of the page is correct """ driver.get("https://qxf2.com/selenium-tutorial-main") expected_title = "Qxf2 Services: Selenium training main" assert expected_title in driver.title, f"Expected title: {expected_title}, but got: {driver.title}" driver.quit() |
b. When you run the test above, it will execute using the default Chrome browser installed on your system. However, our goal is to run it using the Replay browser. To do this, we need to figure out the location of Replay browser’s binary file. You can find it in the following directory within your Replay installation: .replay/runtimes/chrome-linux/chrome. The absolute path would look similar to this: /home/ubuntu/.replay/runtimes/chrome-linux/chrome.
c. Next, we need to set this path as the browser’s binary location in our test. We can do this by setting the Chrome driver options using the Chrome 'Options'
class.
"""' This is a simple test to demonstrate integration of Replay with Selenium """ from selenium import webdriver from selenium.webdriver.chrome.options import Options # Set the Chrome driver options options = Options() options.binary_location = "/home/ubuntu/.replay/runtimes/chrome-linux/chrome" driver = webdriver.Chrome(options=options) def test_title_selenium_tutorial(): """ This is an sample automated test to check if the title of the page is correct """ driver.get("https://qxf2.com/selenium-tutorial-main") expected_title = "Qxf2 Services: Selenium training main" assert expected_title in driver.title, f"Expected title: {expected_title}, but got: {driver.title}" driver.quit() |
In the above code, we have used options.binary_location
to set the location of the Chrome binary that selenium uses.
d. Your tests should now run against the Replay browser. However, it wouldn’t record anything yet.
3. Key step: Enable recording the browser Session
By default, the Replay browser does not record your browser session. To enable it, we need to set the environment variable RECORD_ALL_CONTENT
to 1
.
export RECORD_ALL_CONTENT=1 |
Once enabled, run the test again. It should now record your browser session. To view the recordings you can run the following command:
replayio list |
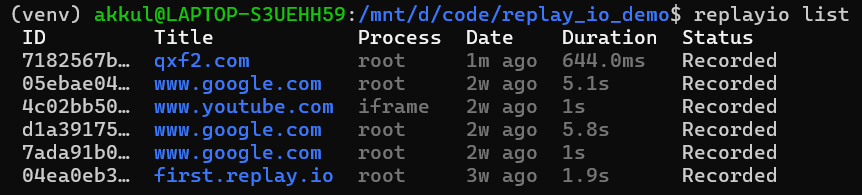
4. Uploading and debugging the replay
To upload your replay to the Replay.io server, you can run the following command:
replayio upload <recording-id> |
This will upload your replay to the Replay.io server and provide you with a link to the recording. You can then examine and debug the replay as per your requirements.
5. When to Use Replay
Now, let’s take a look at some scenarios where Replay can improve your testing process:
a. Complex Debugging: If you’re dealing with complex issues that are difficult to reproduce, Replay is an excellent tool. It captures all interactions, making it easier to record and reproduce bugs that may not be apparent in a single test run.
b. Collaboration: Replay allows you to share replays with team members, enabling collaboration and easier communication of issues.
6. Where to Avoid using Replay
Lastly, let’s look at certain scenarios where it would be better to avoid using Replay.
a. Tests with Sensitive Data: If your tests interact with sensitive or personal data, be cautious about using Replay. The tool captures all browser interactions, which could inadvertently include the sensitive information. Ensure that you’re compliant with your organization’s data protection policies before using Replay in such scenarios.
b. Browser-Specific Tests: If your tests are designed to verify functionality in a particular browser environment or rely on browser-specific features, it may be better to avoid using Replay. Replay might not accurately represent the unique behaviors or quirks of specific browsers.
We hope this blog has provided valuable insights into setting up Replay.io with Python Selenium. If you have any questions or need further assistance, feel free to reach out. Happy testing!
Hire testers from Qxf2
Qxf2 employs highly technical software testers. We help early stage companies setup their QA function, lay the foundation for good testing, kickstart test automation, augment existing teams and so much more. If you are looking for experienced testers with strong technical capabilities, reach out to us.
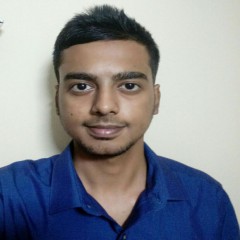
I am a QA Engineer. I completed my engineering in computer science and joined Qxf2 as an intern QA. During my internship, I got a thorough insight into software testing methodologies and practices. I later joined Qxf2 as a full-time employee after my internship period. I love automation testing and seek to learn more with new experiences and challenges. My hobbies are reading books, listening to music, playing chess and soccer.