Problem: There is not much information on how to send or attach images to ReportPortal
Why this post?
We started using ReportPortal for reporting automation test results. Along with the test results we also need to send log messages and images/screenshot to ReportPortal. These are useful artifacts which help people monitoring them check the success and failure reasons and take necessary actions.
We were not able to find information on how to send a screenshot to ReportPortal easily and had to spend some time to get it going. So this blog post is to share our knowledge on how to send the images to ReportPortal using pytest.
Note: This is in continuation to our previous post on ReportPortal integration with pytestnd pytest markers
How to send logs and images to ReportPortal
1. Sending log messages
The agent-python-pytest plugin provides a python logging handle which can be used in conftest.py file.
@pytest.fixture(scope="session") def rp_logger(request): import logging # Import Report Portal logger and handler to the test module. from pytest_reportportal import RPLogger, RPLogHandler # Setting up a logging. logging.setLoggerClass(RPLogger) logger = logging.getLogger(__name__) logger.setLevel(logging.DEBUG) # Create handler for Report Portal. rp_handler = RPLogHandler(request.node.config.py_test_service) # Set INFO level for Report Portal handler. rp_handler.setLevel(logging.INFO) return logger |
We can then use the rp_logger in tests to send log messages easily.
def test_launch_success(rp_logger): rp_logger.info("A GUI test. Launch Qxf2.com") |
2. Sending screenshot
We can use the same method rp_logger along with the attachment details to send image to ReportPortal. We need to pass the image_name which will be the name of the image in ReportPortal, data which is the image and the mime type.
rp_logger.info( image_name, attachment={ "data": image, "mime": "application/octet-stream" }, ) |
We have created a separate method save_screenshot_reportportal() which takes in an image and sends it to ReportPortal. We also need to open the .png(binary file) before sending the image file.
Below is the complete code for the test file.
# Sample test to load Qxf2.com and send image to ReportPortal import pytest from selenium import webdriver @pytest.mark.gui_test() def test_launch_success(rp_logger): "Launch Qxf2 and save image" # Log message to ReportPortal rp_logger.info("GUI Test. Launch Qxf2.com") driver = webdriver.Chrome() driver.get("https://qxf2.com") image_name = "Test_Launch_Success" driver.save_screenshot(image_name+'.png') # Save screenshot to ReportPortal save_screenshot_reportportal(rp_logger,image_name) def save_screenshot_reportportal(rp_logger,image_name): "Method to save image to ReportPortal" try: with open(image_name+'.png', "rb") as fh: image = fh.read() rp_logger.info( image_name, attachment={ "data": image, "mime": "application/octet-stream" }, ) except Exception as e: print("Exception when trying to save screenshot to reportportal: %s" %str(e)) @pytest.mark.api_test() def test_api(): print ("API Test") pass |
3. Running the test
We can use the same conftest file mentioned in the previous post by adding the rp_logger method mentioned in step 1 and run the test
There we go, we now have our screenshots and log messages attached in ReportPortal. Enjoy testing and reporting…
References:
1. ReportPortal python client
2. agent-python-pytest
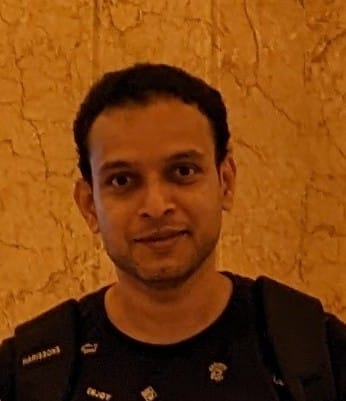
I am a dedicated quality assurance professional with a true passion for ensuring product quality and driving efficient testing processes. Throughout my career, I have gained extensive expertise in various testing domains, showcasing my versatility in testing diverse applications such as CRM, Web, Mobile, Database, and Machine Learning-based applications. What sets me apart is my ability to develop robust test scripts, ensure comprehensive test coverage, and efficiently report defects. With experience in managing teams and leading testing-related activities, I foster collaboration and drive efficiency within projects. Proficient in tools like Selenium, Appium, Mechanize, Requests, Postman, Runscope, Gatling, Locust, Jenkins, CircleCI, Docker, and Grafana, I stay up-to-date with the latest advancements in the field to deliver exceptional software products. Outside of work, I find joy and inspiration in sports, maintaining a balanced lifestyle.
Hi,
This is very useful post .I facing an error when try to use rp_logger in other class inside method directly and getting an errot that attribute is not defined
Nagarjuna, You can configure rp_logger as a global variable.
Not sure if some can help same for JAVA+TEsngNG.I am getting hard time in sending screenshot to reportportal.
Hi,
I am not sure you have tried this link. If not you can refer this link. I hope it should be helpful for you.
“https://github.com/reportportal/example-java-TestNG”
Hi , Looks like this link is broken
Hi,
Can anybody help with Sending screenshots to rportportal for Java + TestNG .
Hi,
If you have not tried this already, Please refer to https://github.com/reportportal/agent-java-testNG
Anybody got the issue resolved??
Im getting this – com.epam.reportportal.message.ReportPortalMessage@1d0305fb
Using version – implementation ‘com.epam.reportportal:logger-java-log4j:5.1.3’
Hi,
We have blogged our experience of using pytest plugins to send images and log messages to ReportPortal. However, We would want to try and help if you are using other frameworks/languages. Could you provide enough details about the issue you are facing?
Regards,
Preedhi
How to see the report after running the tests ? Can someone please guide
Hello,
Did you check our other blog Report portal Integration with pytest and pytest markers
here is the link : https://qxf2.com/blog/reportportal-integration-with-pytest/
Let us know if there are any further questions.
Thanks,
Raghava