At Qxf2 , we provide tips and tricks to make testing more efficient and smoother. This post documents the switch_page functionality of Qxf2’s Page Object Model. Our framework uses a PageFactory class to interface the tests and modeled pages. When the automation wants to interact with a new page of the application, it can simply ask PageFactory for the new page.
This approach, in general, is good. But it can be optimized in some cases. Specifically, if you know that the application has moved to a new page because of a certain action (e.g.: clicking a link), you can simply call a switch_page() method. It magically changes your test_obj’s class to be that of the newly navigated page.
How switch_page() works:
Base page has a method called switch_page() that is intended to be used in this page object model automation testing framework. The method takes one argument page_name, which is the name of the page that the underlying class should be switched to.
The method uses the PageFactory class to get an instance of the required page object. The PageFactory class is responsible for creating an instance of the appropriate page class based on the page_name provided.
In summary, this method allows you to switch the underlying class of the current object to a different page object, making it easier to navigate between pages while using this page object model framework.
Wait! Why do we need this method?
Underneath, switch_page() calls PageFactory. So why do we need switch_page in the first place? Couldn’t we just get the testers to call PageFactory in these methods? Well, we could. But switch_page acts like syntactic sugar for the same functionality. For those interested, switch_page() works on the dunder class member of the test_obj. It’s implementation within our Base Page looks like this:
def switch_page(self,page_name): "Switch the underlying class to the required Page" self.__class__ = PageFactory.PageFactory.get_page_object(page_name,base_url=self.base_url).__class__ |
Guidelines to use switch_page
We suggest you use switch_page keeping these guidelines in mind.
1. switch_page() should be used when a user interaction causes the application to transition from one page to another
2. Use it within a modeled page or modeled page object method
3. Be careful to verify that the page transition happened before you call switch_page
That’s about it. Folks who wanted a high level overview of switch_page() functionality can stop reading here.
For folks who want a practical implementation example, read on.
Implementation Examples
We are taking example of our weather-shopper app here to explain the switching of pages through page factory and the switch page method.
Here is the workflow that we would be testing in the same
a) Visit the main page
The test starts by visiting the main page of the Weathershopper app.
b) Get the temperature
The test then reads the temperature from the main page.
It identifies and extracts the numeric value of the temperature displayed on the webpage.
c) Choose to buy sunscreen or moisturizer
Depending on the temperature reading:
If the temperature is greater than 34 degrees:
The test decides to buy sunscreen.
If the temperature is 19 degrees or lower:
The test decides to buy moisturizers.
d) Add products based on min-max logic:
After deciding whether to buy sunscreen or moisturizers, the test navigates to the respective product type page.
On the product type page, the test adds products to the cart based on a logic.
Test will add the cheapest and most expensive (min and max of price value) of sunscreen or moisturizer available in the product page.
e) Verify the cart
Finally, the test verifies the contents of the shopping cart.
In cart, we will verify the contents of the cart, calculate the total price and validate against the total displayed.
Returning page objects directly
At the beginning of the tests, we will work directly with the page objects.
#Create a test object for the weathershopper main page test_obj = PageFactory.get_page_object("Main Page",base_url=base_url) |
Weathershopper main page will be handling visiting the main page and get the temperature.
Now test will validate the temperature for which product type(depends on the temperature logic)
product_type = "" if temperature <= 19: product_type = "moisturizers" if temperature >= 34: product_type = "sunscreens" result_flag = test_obj.click_buy_button(product_type) |
switch_page() method
Now comes the time to interact as a user, if the product selected is moisturizers or sunscreens, click on the Buy button near to the product_type.
We will call this method from the test.
However, the test object in the Weathershopper main page will perform the method with the logic as follows
def click_buy_button(self,product_type): "Choose to buy moisturizer or sunscreen" result_flag = False product_type = product_type.lower() if product_type in ['sunscreens','moisturizers']: result_flag = self.click_element(self.BUY_BUTTON%product_type) self.conditional_write(result_flag, positive="Clicked the buy button for %s"%product_type, negative="Could not click the buy button for %s"%product_type) result_flag &= self.smart_wait(5,self.PAGE_HEADING%product_type.title()) if result_flag: self.switch_page(product_type) |
This code defines a method that simulates a user clicking the buy button for a specific type of product, waits for the new page to load, and switches to the new page.
Now in the next scenario, we would be adding the products with certain logic(min and max price) and then from the test, action should navigate to the cart page of an e-commerce website or application and logs the result of this action.
#Go to the cart result_flag = test_obj.go_to_cart() test_obj.log_result(result_flag, positive="Automation is now on the cart page", negative="Automation is not on the cart page", level="critical") |
Then Product page test object will have a method defined for the switch page action by using switch page method. The go_to_cart() method provides a convenient way to navigate to the cart page and verify that it has been displayed correctly. If either the button click or the page verification fails, the method will return False, indicating that the operation was not successful.
def go_to_cart(self): "Go to the cart page" result_flag = self.click_cart_button() result_flag &= self.verify_cart_page() |
In the same Product Page, will define the methods to click and verify the cart. These methods can be used in automated tests to simulate user interactions with the cart button and verify that the user is on the cart page.
def click_cart_button(self): "Click the cart button" result_flag = self.click_element(self.CART_BUTTON) self.conditional_write(result_flag, positive="Clicked on the cart button", negative="Could not click on the cart button") return result_flag def verify_cart_page(self): "Verify automation is on the cart page" result_flag = self.smart_wait(5,self.CART_TITLE) self.conditional_write(result_flag, positive="Automation is on the Cart page", negative="Automation is not able to locate the Cart Title. Maybe it is not even on the cart page?") if result_flag: self.switch_page("cart") return result_flag |
Conclusion
In summary, whether opting for a switch_page() or directly interacting with page objects, both approaches contribute to an effective testing strategy, depends on the scenario that we need to test. The “switch_page()” method, involves a user interaction and then transition from one page to another(click of a button, click on link or image). The direct return of page object, this means that when initiating a test, the focus is on interacting directly with the page objects. We could call any page object at anytime(may not be in the user flow).
Hire QA from Qxf2
Qxf2 offers a range of testing services. While we primarily cater to the early stage products and highly technical testing, our recent offerings have expanded to staff augmentation and UI/API test automation. We have tasted success using generative AI methods to produce UI and API in the style of Qxf2’s test automation framework quickly. If you are looking to kickstart your automation efforts and see results quickly, get in touch with us.
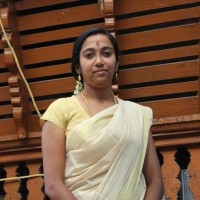
I started my career in IIIT Bangalore incubated startup company as a software engineer and soon started working on digital and e-learning solutions as QA. Later moved to Oracle as User Assistance QA Engineer and gained multiple Oracle cloud products and testing skills. Oracle gave opportunities with products, courses, and environments to explore and enhance testing skills. Now joined Qxf2, to explore in testing perspective. I believe Quality means doing it right, even when no one is looking. A number of bugs are not evaluating the product, the quality of the bug also matters. I love cooking, reading, and DIY crafts.